REST API 란?
REpresentional State Transfer의 약자로서, 어떤 자원에 대해 CRUD를 진행할 수 있게
HTTP Method(GET, POST, PUT, DELETE)를 사용하여 요청을 보내는 것.
이 때, 요청을 위한 자원은 특정한 형태로 표현된다.
# CRUD - Create, Read, Update, Delete 를 처리하는 동작
간단하게 : "URI를 통해 정보의 자원을(only 자원만을) 표현하고, 자원의 행위는 HTTP Method로 명시한다.
# 규칙
http://example.com/posts (O)
http://example.com/posts/ (X)
http://example.com/post (X)
http://example.com/get-posts (X)
--> URI는 명사를 사용하고 소문자로 작성되어야 한다.
--> 명사는 복수형을 사용한다.
--> URI의 마지막에는 /를 포함하지 않는다.
http://example.com/post-list (O)
http://example.com/post_list (X)
--> URI에는 언더바가 아닌 하이픈을 사용한다.
http://example.com/post/assets/example (O)
http://example.com/post/assets/example.png (X)
--> URI에는 파일의 확장자를 표시하지 않는다.
RestFul 하다 - 누가봐도 이해하기 쉽고, 위 조건을 만족 시킨 상태
Path Variable vs Query Parameter
- Path Variable
/users/10
- 유츄 가능. 전체 데이터 or 특정 데이터를 다룰 때 처럼, 리소스를 식별하기 위해 씀 - Query Parameter
/users?user_id=10
- 데이터를 정렬하거나 필터링 하는 경우 더 적합
JSON ( JavaScript Object Notation )
자바스크립트 객체 문법에 토대를 둔, 문자 기반의 데이터 교환 형식
구조 :
일반적인 JSON 구조는 JS 객체 리터럴 작성법을 따름.
JS의 원시 자료형인 문자열, 숫자, 불리언을 가질 수 있고 중첩된 계층 구조 또한 가질 수 있다.
메서드 :
JSON -> 문자열 형태 -> 서버 - 클라이언트 간 데이터 전송 시 사용
but, 다음 두 경우를 위해 파싱(parsing)과정이 필요.
1. JS 객체를 JSON 형태로 전송
2. JSON 형태를 JS 객체 형태로 사용
# stringify()
: JS 객체 -> JSON 문자열 변환. 네트워크를 통해 JSON 문자열로 변환할 때 주로 사용
console.log(JSON.stringify({ x: 5, y: 6 }));
// Expected output: "{"x":5,"y":6}"
console.log(JSON.stringify([new Number(3), new String('false'), new Boolean(false)]));
// Expected output: "[3,"false",false]"
console.log(JSON.stringify({ x: [10, undefined, function(){}, Symbol('')] }));
// Expected output: "{"x":[10,null,null,null]}"
console.log(JSON.stringify(new Date(2006, 0, 2, 15, 4, 5)));
// Expected output: ""2006-01-02T15:04:05.000Z""
# parse()
: JSON 문자열 -> JS 객체 변환. 네트워크 통신의 결과를 통해 받아온 JSON 문자열을
프로그램 내부에서 사용하기 위해 JS 객체로 변환할 때 사용
const json = '{"result":true, "count":42}';
const obj = JSON.parse(json);
console.log(obj.count);
// Expected output: 42
console.log(obj.result);
// Expected output: true
# JSONPlaceholder
: 가짜 서버(= fake server, mock API server)
애래 API를 통해 JSON 기반 DB 통신을 일으켜보기
fetch('https://jsonplaceholder.typicode.com/posts')
.then((response) => response.json())
.then((json) => console.log(json));
App.jsx
import "./App.css";
import { useEffect, useState } from "react";
function App() {
const [data, setData] = useState([]);
useEffect(() => {
fetch("https://jsonplaceholder.typicode.com/posts")
.then((response) => {
console.log();
console.log("response.json()", response);
return response.json();
})
.then((json) => {
console.log("json", json);
setData([...json]);
});
}, []);
return (
<div>
{data.map((item) => {
return (
<div
style={{
border: "1px solid black",
margin: "3px",
}}
key={item.id}
>
<ul>
<li>userId : {item.userId}</li>
<li>id : {item.id}</li>
<li>title : {item.title}</li>
<li>body : {item.body}</li>
</ul>
</div>
);
})}
</div>
);
}
export default App;
결과
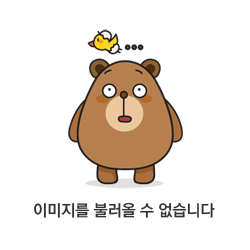
'React > 1' 카테고리의 다른 글
나만의 React App 만들기 (1) | 2024.11.02 |
---|---|
비동기 프로그래밍 (0) | 2024.11.02 |
react-router-dom (2) | 2024.11.02 |
why React (0) | 2024.11.02 |
Redux (0) | 2024.11.01 |